- Before you build your mini game on WeChat, read this!
- Get to know the WeChat mini game infrastructure
- Introduction On The WeChat SDK
Introduction
In the last two articles "Before You Build Your Mini Game On WeChat, Read This!," and "Get To Know The WeChat Mini Game Infrastructure" we introduced everything you needed to get started with WeChat mini game development, as well as the development environment and tools of a WeChat mini game.
Today we will analyze in more detail the various SDK interfaces that the mini game environment has and what kind of gaming experience these interfaces can carry. Of course, in the official WeChat game documentation, there are very detailed introductions to these interfaces. We won't share the details about the API calls but will focus more on the functions and potential provided by these interfaces. Finally, we will share how you can use Cocos Creator in making mini games.
The Interface Capabilities Of The Mini Game
WeChat SDK Interface General Rules
Most of WeChat's SDK interfaces have very closed usage and naming rules. Here are some of the experiences we have using it:
▪ on??? & off???: This type of API is generally used for event registration and de-registration.
▪ ???Sync: Adding Sync after a function name makes it a synchronization method of the function, and also indicates that the original function must be called asynchronously.
▪ Asynchronous functions: Since many WeChat APIs need to make background requests or get information from WeChat running kernel, there are a large number of asynchronous interfaces, sometimes providing synchronous versions of them. However, in most cases, we still recommend using asynchronous interfaces. To make it easier to catch exceptions and organize exception handling code.
▪ Calling asynchronous functions: Most asynchronous functions in the WeChat API accept an object as a parameter, which should contain:
success: successful callback
fail: failure callback
complete: complete callback (call will succeed if executed successfully)
wx.getSystemInfo({
success: function (res) {
// the object containing the result of the call
console.log('This phone is ' + res.brand + ' ' + res.model);
},
fail: function (res) {
// get the error message with res.errMsg
console.warn(res.errMsg);
},
complete: function () {
console.log('API call completed');
}
})
▪ get??? & set???: Get and set the interface. It is counter-intuitive that many of these interfaces are also asynchronous, so you need to read the API documentation carefully.
Next, let's look at the three major interfaces that everyone cares about most: users, forwarding, and payment interfaces.
User Interface
In terms of a user interface, the most important thing developers should pay attention to is the user's login. The usage examples of the login interface are as follows:
wx.login({
success: function (res) {
// the object containing the result of the call
if (res.code) {
// Game server handles user login
} else {
// handle any failure
console.log('Failed to get user login status!' + res.errMsg);
}
},
fail: function (res) {
// handle any failure
console.log('user login failed' + res.errMsg);
}
});
As mentioned above, to achieve a good user experience, all asynchronous interface failures should be processed. Login is even more so because if Login fails, the game can't proceed unless it is a pure stand-alone game. As for the failure process, we recommend retrying or guiding the user to close the game and try again.
The res.code in the callback interface is the user's login credentials, which can be used to exchange information such as OpenID and session_key in the background of the developer server. Some APIs may contain sensitive data of the user. This sensitive data needs to be passed by session_key to obtain. Otherwise, you'll only get the minimal basic data. For details, please refer to the user login status signature document. Here are the APIs that are required to get a login status signature to obtain sensitive data is:
User login signature document: https://developers.weixin.qq.com/miniprogram/en/dev/
- wx.getUserInfo: Get user information
- wx.getShareInfo: Get forwarding details
- wx.getWeRunData: Get WeChat pedometer information
Also, some API calls require the user's authorization to use. If no approval has been applied, the first call will be automatically applied. The process is as follows:
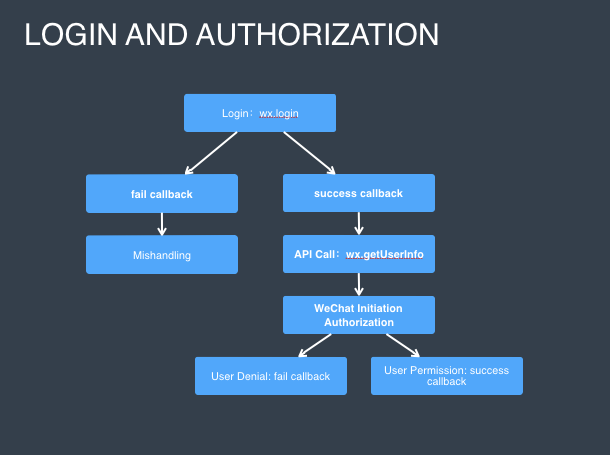
You can also pre-authorize before calling the API:
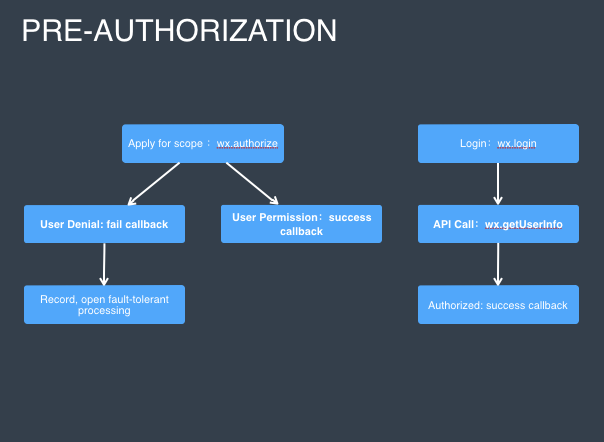
For a detailed list of authorizations and a list of APIs that require an authorization, please refer to the User Authorization section in the official WeChat document at:
https://developers.weixin.qq.com/miniprogram/en/dev/framework/open-ability/authorize.html
Forwarding interface
In the first article, we mentioned the most prominent ability of mini game, is the ultra-fast experience of directly entering the game from the app. From a technical point of view, forwarding information in a mini game is divided into passive forwarding and active forwarding (active and passive for game developers):
Passive Forwarding
Use wx.showShareMenu to display the forwarding options in the pop-up menu of the "..." button in the upper right corner so that forwarding can be initiated at any time during the user's game. The forwarding option can be removed via wx.hideShareMenu.
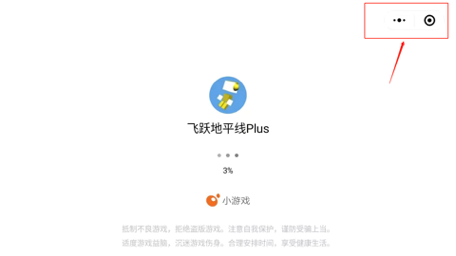
At the same time, developers can listen to wx.onShareAppMessage to listen for user forwarding behavior and prepare appropriate forwarding content. Specifically, the developer can customize the forwarding content in the return value of the callback function:
- title: title, if not passed, the nickname of the current mini game is used by default.
- imageUrl: Forward an image link to display the image.
- query: Game parameters, follow the query string in the format of key1=val1&key2=val2. After entering this forwarding message, these startup parameters can be obtained through wx.onLaunch or wx.onShow.
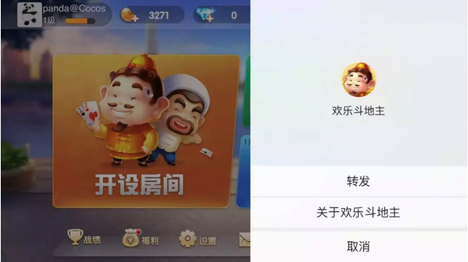
right corner to initiate the forwarding.
Active Forwarding
Active forwarding means that the developer actively initiates a forwarding request for the user in the game interaction. Generally, after the player clicks a share button in the game, the developer directly calls the wx.shareAppMessage to transfer the forwarding window.
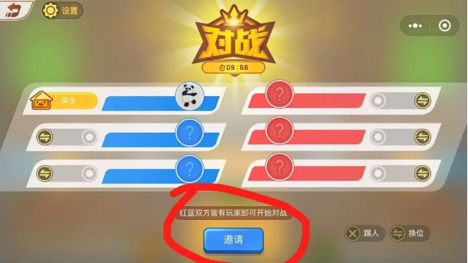
Developers can also set the withShareTicket mode for all forwarding. In this mode, the developer can get a shareTicket when forwarding and entering the game through the forwarding link. Pass the shareTicket into wx.getShareInfo to decrypt the data in the callback to get the shared group id.
For data decryption, please refer to the encryption and decryption algorithm documentation at https://developers.weixin.qq.com/miniprogram/en/dev/quickstart/basic/file.html
Payment System
The Payment system comes with two calls: wx.requestPayment to ask for the payment and wx.chooseAddress to request the shipping address of the user for those mini apps that send items to a user. This isn't a necessary item.
Network Interface
The network interface is divided into three parts: network request, WebSocket, upload, and download.
Network request
The API of the network request is wx.request. This interface can be understood as the XMLHttpRequest in the browser. In fact, the XHR object in the Adapter is also used to encapsulate it. It can be used to make network requests and get the data returned by the remote server. It is worth mentioning that this data is stored in memory and can be used directly by the user without any file IO operations.
WebSocket
The use of WebSocket related interfaces can be referred to as the API documentation. Although the interface definition is not the same as in the browser, the Adapter provides a new interface based on the WeChat based API. So you can directly use the Adapter script, eliminating the need for adaptation. Of course, if the network request in the game encounters a problem, you may need to debug and locate it through the original API.
Upload / Download
WeChat also provides direct upload (wx.uploadFile) and download (wx.downloadFile) APIs that are not available in the browser.
For game development, we need to focus on downloading the API. First of all, it is different from the purpose of the request. The request is used to request various forms of data, including strings, file data, ArrayBuffer, JSON objects, and so on. So it is suitable for short link requests, Restful API, and wx.downloadFile to directly download files to the local device.
If you specify the filePath in the call parameter object, the downloaded file will be stored in the local cache space of the game, otherwise the downloaded file will be stored in a temporary space, and it will be deleted when you exit the game, depending on whether you need to keep this file for a long time. However, it should be noted that if the file path has a relative path and the path does not exist in the local cache, the download will fail. This requirement must be met with the file system API.
File System
Mini games have a resourceful file system interface for developers, which is entirely different from the case where file IO is not supported in the browser. On the one hand, this gives developers more freedom and space, but on the other hand, because the WeChat game environment does not support browser-like resource caching and resource expiration mechanism, it's necessary to use the API for the WeChat game environment.
Specifically, the browser caches the resources that the user has already accessed. When it is reaccessed, it will obtain the priority from the cache instead of sending the request to the server. This can reduce network usage and optimize the page response speed. When the server resource is updated, the browser will find that the local resource has expired, automatically clear the corresponding local resource and get the latest version from the server.
In a mini game environment, if you want to avoid getting resources from the server every time, you need to implement a similar resource cache and expiration scheme. Such a program has to rely on the above download interface and file system interface. The good news is that the Cocos Creator provides a complete resource management solution that we will discuss in detail in the future.
To understand the file system of a mini game, first understand the file sandbox environment of the mini game:
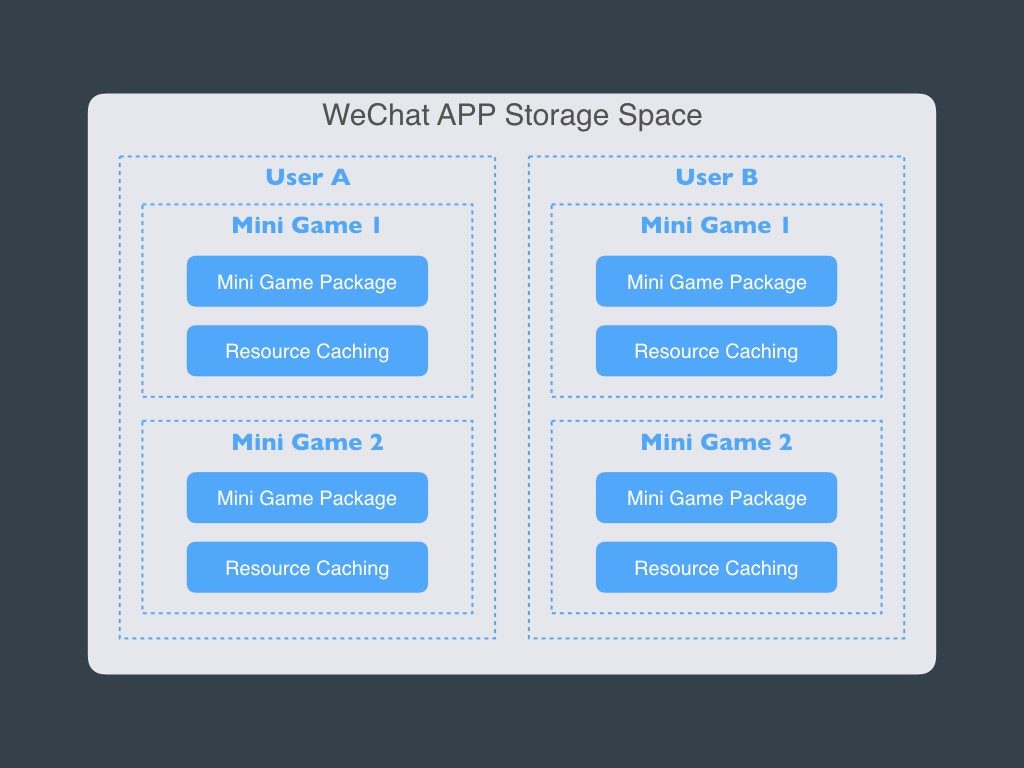
All file system interfaces are executed in this file's sandbox environment. All file directories are also relative to the sandbox environment, so we don't have to worry about file conflicts between different games or different users.
From the perspective of API usage, all file system interfaces are provided by the FileSystemManager, and developers need first to pass:
Let fs = wx.getFileSystemManager();
To get the FileSystemManager object and then call its API to complete the required functions, the following shows the usage of the API by downloading, reading, and deleting the file flow:
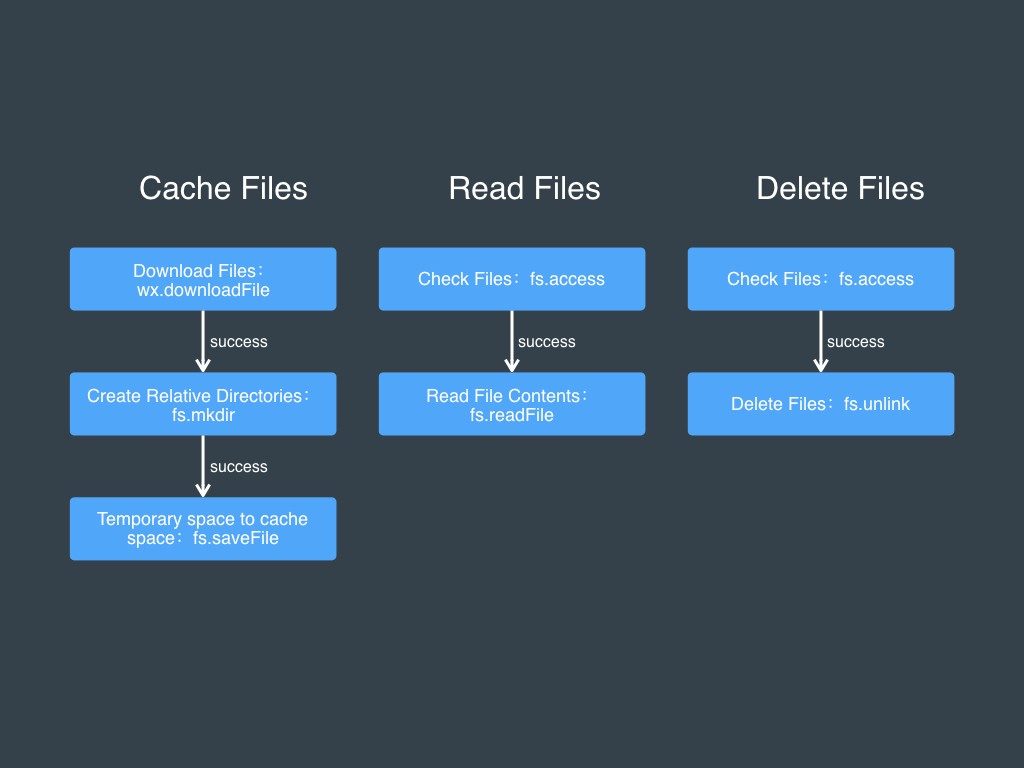
These are just some of the most basic interfaces used. Also, WeChat games provide renameFile, copyFile, readdir, writeFile, etc., and you can refer to the API documentation to explore. Attentive developers will also notice that most of these interfaces contain synchronous versions, such as fs.readFileSync. We recommend using an asynchronous version of the interface. Otherwise, the blocking caused by file IO will affect the smoothness and play experience of the game.
Other Interfaces
In addition to these interfaces, here are some examples of essential interfaces for WeChat mini game:
▪ wx.getSystemInfo: Get system information, including mobile phone brand, model, screen width, language, version, power, and other information.
▪ wx.onAccelerometerChange: monitors accelerometer events and can be used for control input of some games.
▪ wx.setKeepScreenOn: Keep the screen always on.
▪ wx.vibrateShort: 15ms short vibration, there is also an extended vibration interface, which can be used to enhance the game experience.
▪ wx.getLocation: Get the geographic location, speed, etc., LBS function is required.
▪ Keyboard interface: Contains a display/hide keyboard and various keyboard input listener interfaces that can be used to create input boxes in the game. The Cocos Creator has already packaged this into the EditBox component in the same way as the browser environment.
▪ wx.getRecorderManager: Get the recording manager, which can be used to enter the player's voice.
▪ Picture interface: used to select pictures from the user's albums, or save photos to the user's albums.
▪ wx.createVideo: Create a video, provide video playback, and a video control interface.
▪ wx.triggerGC: If the WebGL texture is frequently created and destroyed in the game, or the memory usage is too high, you need to call this interface to trigger the GC at the appropriate time to reduce the game memory usage.
Make Your First Mini Game With Cocos Creator
Now that you have a better understanding of the API and SDK, you might feel it's a bit tough to get your game can work in WeChat. However, this isn't a problem with the reliable support that a game engine can provide. As Cocos Creator runs JavaScript and has been working with Tencent to improve compatibility, we are one of a few engines tailor-made to help you get your mini game into WeChat. If you want to start building your game. If you're going to use Cocos Creator for your next WeChat mini game, follow these steps:
▪ First download Cocos Creator v2.1.2 for either Windows or Mac. (Starting with version 1.8, we officially support the release of mini games.)
▪ After the installation is complete, open Cocos Creator to the Dashboard panel, select the new project, select the Hello World project template, set the project path, and click "New Project" to create.
▪ After opening the project, you can open the "Scene/HelloWorld" scene, and you can make some modifications if you are interested.
▪ Find the "Cocos Creator > Preferences" option from the menu bar and open it, click on the "Native Development Environment" setting, set the installation path of the WeChat Developer tool in the WechatGame program path, and finally, click "Save and Close."
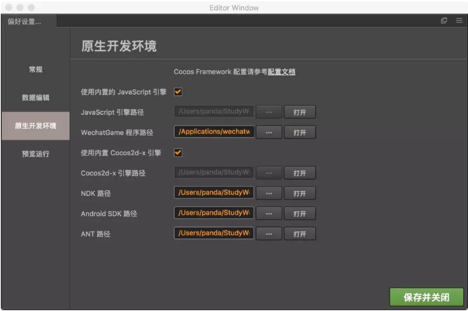
▪ From the menu bar, find the "Project > Build…" option and open it. In the Build Publishing panel, select the publishing platform as Wechat mini game, set the device orientation, appid, debug mode, etc. Finally, click "Build." (If you don't have your game appid, you can use the visitor appid: wx6ac3f5090a6b99c5).
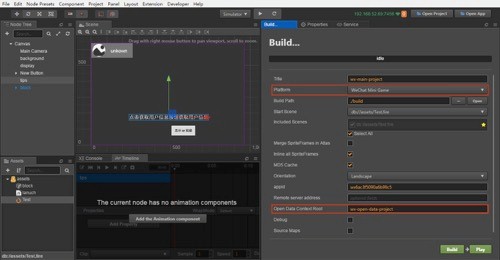
▪ When the progress bar above the build release panel is finished, and sleep is displayed in the log, it means the build is complete. At this point, click play to automatically adjust to the WeChat developer tool and view the correct scene effects. (If you don't automatically open the WeChat Developer tool, or don't automatically open the game project, you can use the WeChat Developer tool to create a small game experience project in the project directory under the build/wechatgame project path).
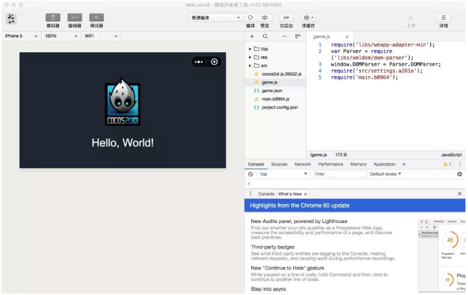